 |
|
 |
|
eXercise #15
Compound Data Structures
- The ARRAY
Vectors - One Dimensional
Arrays
- Given the definitions
and declarations
const Last-position = 30;
type Indices = 1.. last_position;
Whole = 0..maxint;
Letters = 'A'..'Z';
Messages = array [ indices] of char;
Distributions = array [letters] of whole;
var Message : messages;
FrequencyOf : distributions;
and the assignment
Message := 'THIS IS A SAMPLE LINE OF TEXT.'
- Write a function
called SpacesIn which scans the Message vector and returns
the number of spaces.
- Write a small
fragment of code which sets every element of the vector FrequencyOf
to zero.
- Write a procedure
called Scan which scans the character vector Message and
counts the number of times each letter appears in the vector.
- Write a Function
called HowMany, that accepts a character and returns the
number of times that character appears int he message
Test this procedure, HowMany('A') should return the value 2 because
there are two 'A's in Message, while HowMany('B') should return
the value 0 because 'B' does not appear in Message.
- Using the
FrequencyOf array, store the number of each letter of the alphabet
that occurs in the message (FrequencyOf[1] should store the number
of 'A's, FrequencyOf[2] should store the number of 'B's and so
on) - try to be as efficient as possible in this tallying process.
Declare whatever additional variables you require.
- Write a procedure
called PlotDistribution which scans the vector FrequencyOf and
which draws a bar graph of asterisks (representing the numbers
stored there) as follows.
A **
B
C
D
E ***
F *
:
- Write a program
which prints out the first 13 rows of Pascalls triangle. For your
reference, the first few rows are:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Strings - One Dimensonal
Arrays of Char
- Given the declarations
var reply : string[80];
1, n : integer;
r : real;
and the assignment
reply := 'the albatross did not signify'
determine the value
of
- reply [1]
- ord (reply[0])
- reply [length(reply)]
- copy (reply,6,5)
- length (copy
(reply,12,6))
- pos ('ss',
reply)
- copy (reply,19,1)
+ copy (reply,20,2)
- length (reply+reply)
- Use the declarations
and assignment of question 1 to determine the new value of reply after
the application of the following procedures. In each case, assume
that reply has its original value indicated above.
- insert ('I
think 1, reply, 1)
- delete (reply,
22, 7)
- delete (reply,
length(reply), 1)
- insert ('.',
reply, length(reply))
- Assuming the
declarations'of question 1, and the assignment
reply := '12345.6+789'
determine the effect
of the following calls.
- val (copy
(reply,2,4), n, 1)
- val (copy
(reply,4,4), r, 1)
- val (copy
(reply,5,5), r, 1)
- str (17,
reply)
- str (17:3,
reply)
- str (28.74:8:0,
reply)
- Given a string
FRED of current length L, how would you obtain
- the first
n characters of FRED?
- the last
n characters of FRED?
- In each of the
questions which follow, you will be asked to write a procedure which
will augment Pascal's predefined string handling procedures. Note
that, having defined a procedure, you are at liberty to use this in
subsequent procedures. You may assume the following definition and
declarations.
type string8O = string[80];
var S,t,u,a : string80;
i : integer;
Write procedures
which:
- UpShift (s)
Shift any character in a string s to its uppercase equivalent.
e.g.. 'So 16 me' becomes 'SO 16 ME'
- Trim (s)
Remove any leading and trailing spaces from a string s.
e.g., ' Hi there! ' becomes 'Hi there!'
- DeBlank (s)
Remove all blanks from a string s.
e.g., 'Hi there! ' becomes 'Hithere!'
- Collapse
(s) Remove multiple spaces from a string s.
e.g., 'See where the multiple spaces are '
becomes 'See where the multiple spaces are'
- Overwrite(s,t,i)
Substitutes s in string t at position i.
e.g., a := '1961';
overwrite ('73',a,3) a becomes '1973'
- Replace (s,t,u)
Replaces the first occurrence of string s with string t in string
u.
e.g., a := 'chairman';
Replace ('man', 'Person', a)
a becomes 'chairperson'
- ReplaceAll
(s,t,u) Replaces every occurrence of string s with string t in
string u.
e.g., a := 'The chairman's presence was mandatory'
ReplaceAll ('man', 'person', a)
a becomes 'The chairperson's presence was persondatory'
Multi-Dimensioned Arrays
- Noughts and crosses
is a game that uses a grid (in this version, a 4x4 array of char)
with the winner being the first to get '4 in a row'. You can Download
the COMPLETED game by clicking here: tic_tac.exe.
You can download the PARTIAL SOURCE CODE by clicking here : tic_tac.pas
Your mission, Jim, should you chose to accept it, is to copy the partial
source code file to your working directory and then complete the program
so it matches the running one.
EXTRA FOR EXPERTS: the program as given contains many procedures and
functions that are 'impolite' - ie. they clobber GLOBAL variables
without being passed them - re-write the procedures and functions
so they only use objects they either own or were passed. 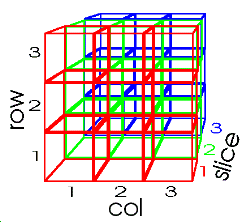
- Three dimensional
Tic_Tac_toe is like it's 2-d counterpart, but more challenging - winning
moves can run horizontally, vertically and diagonally through the
'rubic cube - like space' - your challenge is to write it.
decide first, how to display the workspace - I would suggst you do
so in SLICES. The user will need to enter 3 coordinates to claim a
cell,you will need to validate their choice, then the fun starts -
checking for a win....
Solutions
|
|
|
 |
|
 |
|