 |
|
 |
|
eXercise #20
Some Simple Visual Projects
featuring iteration
index: for..to..do | repeat..until
| while..do
- Cryptography is the art of substituting encoded
symbols for common characters. This project uses a simple displacement
cypher to encode a string.
Imagine a section of the ASCII table, together with the same section
of the ASCII table displaced by a set number of characters:
a
|
b
|
c
|
d
|
e
|
f
|
g
|
h
|
i
|
j
|
k
|
l
|
m
|
n
|
o
|
d
|
e
|
f
|
g
|
h
|
i
|
j
|
k
|
l
|
m
|
n
|
o
|
p
|
q
|
r
|
Because the letters are arranged ordinally in the table, we can 'advance'
them by a fixed amount and therefore seemingly scramble them as shown
below:
In this project, you will construct a form as follows:
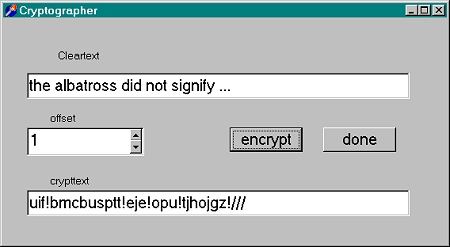
run | cheat
The initial text of each of the edit boxes is largely irrelevant as
eventually the cleartext box will generate the crypttext when the
encrypt button is pressed.
In this cypher - each character has been advanced 1 in the ASCII table:
t becomes u, a becomes b and so on.
The project comprises:
- 1 spin edit box named displacement
- 2 edit boxes called cleartext and crypttext
- an encrypt button and a done button
- some labels for eye-candy value only
Each letter in the cleartext.text can be accessed individually (as
it is a string). The fifth letter, for example is referred to as cleartext.text[5].
You will write the event handler behind the [encrypt] button so that
firstly it correctly converts the first letter (cleartext.text[1])
to it's encrypted form Use ord to turn it into ASCII and char
to convert it back to a char)
The process you use will be generalised and a loop added to step from
the first letter to the last letter, encrypting as you go. We need
a loop starting at 1 and ending at length(cleartext.text) similar
to:
for counter := 1 to length(cleartext.text) do ...
each character of the cleartext can then be accessed by something
similar to: cleartext.text[counter]
The loop should add each encrypted character onto the crypttext (use
the string catenator '+' to do this).
If your program is working correctly, a message encrypted using a
displacement of 3, say, can be decrypted (if you paste the gobbldegook
into the cleartext) using a displacement of -3
- Bang the Weasel.
Construct a form as follows:
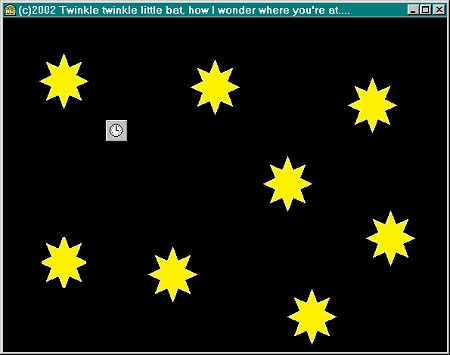
run | cheat
The project (initially) comprises:
Write in the ONTIMER a process that randomly relocates the star
and randomly determines if it is visible or not.
To randomly make an object vanish, you need to set the .visible
property to FALSE. In this program, you could achieve this using
something like:
star.visible := random(100)<50;
To randomly relocate an object, you need to change it's .left
and .top property. In this program, you could change the .top
using something similar to:
star.top := random(clientheight)+1;
The onclick process for the star TImage should be something
simple like close.
*** The POINT of this exercise ***
When you have your SINGLE star working correctly, copy and paste lots
of stars onto the form. Delphi actually keeps track of all the things
on a form using the COMPONENTS array. It is indexed 0..n-1, where
n= the number of components on the form. You can use a loop to cycle
through the things on the form, asking if it is an image, if it is
you then relocate it and randomly make it invisible. A FOR..TO..DO
loop makes most sense to do this.
You can modify your single star process to be smart enough to work
for as many stars as you like by simply including a loop similar to
the following:
for counter := 0 to componentcount do
if (components[counter] is TImage)
then (components[counter] as TImage).top := .....
Experts:
- You should ensure the stars are only randomly located on visible
parts of the form
- Bias the visibility and change the timer speed so it is actually
playable
- You should add some element of game play - the handler that
registers a hit should increment some counter and the game should
terminate only after you kill x stars
- You could add an additional timer that limits the time of the
game - making it necessary to click-frenzy to win before time
runs out.
- CASE STUDY: Hi-Lo Guessing Game
run | cheat
Your Mission, Jim, should you choose to accept it....
Write a program that invents a random number between 1 and 100, then
repeatedly asks the user for a guess reporting either 'Too High' or
'Too Low' until the user gets it right
Guidelines:
- The number should be stored as a BYTE variable
- The prompt for a new guess should be an inputbox (not an edit
box)
- a REPEAT...UNTIL loop should be used here as we are not easily
able to predict how many guesses are necessary, but at least one
is required.
- The progress of the guessing should be displayed somehow as
captions on the form.
Extra for Experts:
- Some track should be kept of the number of goes taken - terminate
the game if more than 10 guesses are taken, say.
- The boundaries could be displayed somehow. If the initial guess
is 50, say, then the program could respond "too high, the
mystery number is between 1 and 50"
- Rework Question 3 using a WHILE..DO instead of the REPEAT..UNTIL
|
|
|
 |
|
 |
|